audio_waveforms
所属分类:Dart语言编程
开发工具:Dart
文件大小:9345KB
下载次数:0
上传日期:2023-05-22 07:17:15
上 传 者:
sh-1993
说明: 使用此插件生成波形,同时以给定编码器支持的任何文件格式或从...
(Use this plugin to generate waveforms while recording audio in any file formats supported by given encoders or from audio files. We can use gestures to scroll through the waveforms or seek to any position while playing audio and also style waveforms)
文件列表:
.metadata (308, 2023-08-14)
CHANGELOG.md (5325, 2023-08-14)
LICENSE (1073, 2023-08-14)
analysis_options.yaml (154, 2023-08-14)
android (0, 2023-08-14)
android\build.gradle (1161, 2023-08-14)
android\gradle.properties (82, 2023-08-14)
android\gradle (0, 2023-08-14)
android\gradle\wrapper (0, 2023-08-14)
android\gradle\wrapper\gradle-wrapper.properties (200, 2023-08-14)
android\settings.gradle (37, 2023-08-14)
android\src (0, 2023-08-14)
android\src\main (0, 2023-08-14)
android\src\main\AndroidManifest.xml (122, 2023-08-14)
android\src\main\kotlin (0, 2023-08-14)
android\src\main\kotlin\com (0, 2023-08-14)
android\src\main\kotlin\com\simform (0, 2023-08-14)
android\src\main\kotlin\com\simform\audio_waveforms (0, 2023-08-14)
android\src\main\kotlin\com\simform\audio_waveforms\AudioPlayer.kt (6535, 2023-08-14)
android\src\main\kotlin\com\simform\audio_waveforms\AudioRecorder.kt (7527, 2023-08-14)
android\src\main\kotlin\com\simform\audio_waveforms\AudioWaveformsPlugin.kt (11531, 2023-08-14)
android\src\main\kotlin\com\simform\audio_waveforms\Utils.kt (2389, 2023-08-14)
android\src\main\kotlin\com\simform\audio_waveforms\WaveformExtractor.kt (9018, 2023-08-14)
example (0, 2023-08-14)
example\.metadata (305, 2023-08-14)
example\analysis_options.yaml (1453, 2023-08-14)
example\android (0, 2023-08-14)
... ...

# Audio Waveforms
Use this plugin to generate waveforms while recording audio in any file formats supported
by given encoders or from audio files. We can use gestures to scroll through the waveforms or seek to
any position while playing audio and also style waveforms.
## Preview
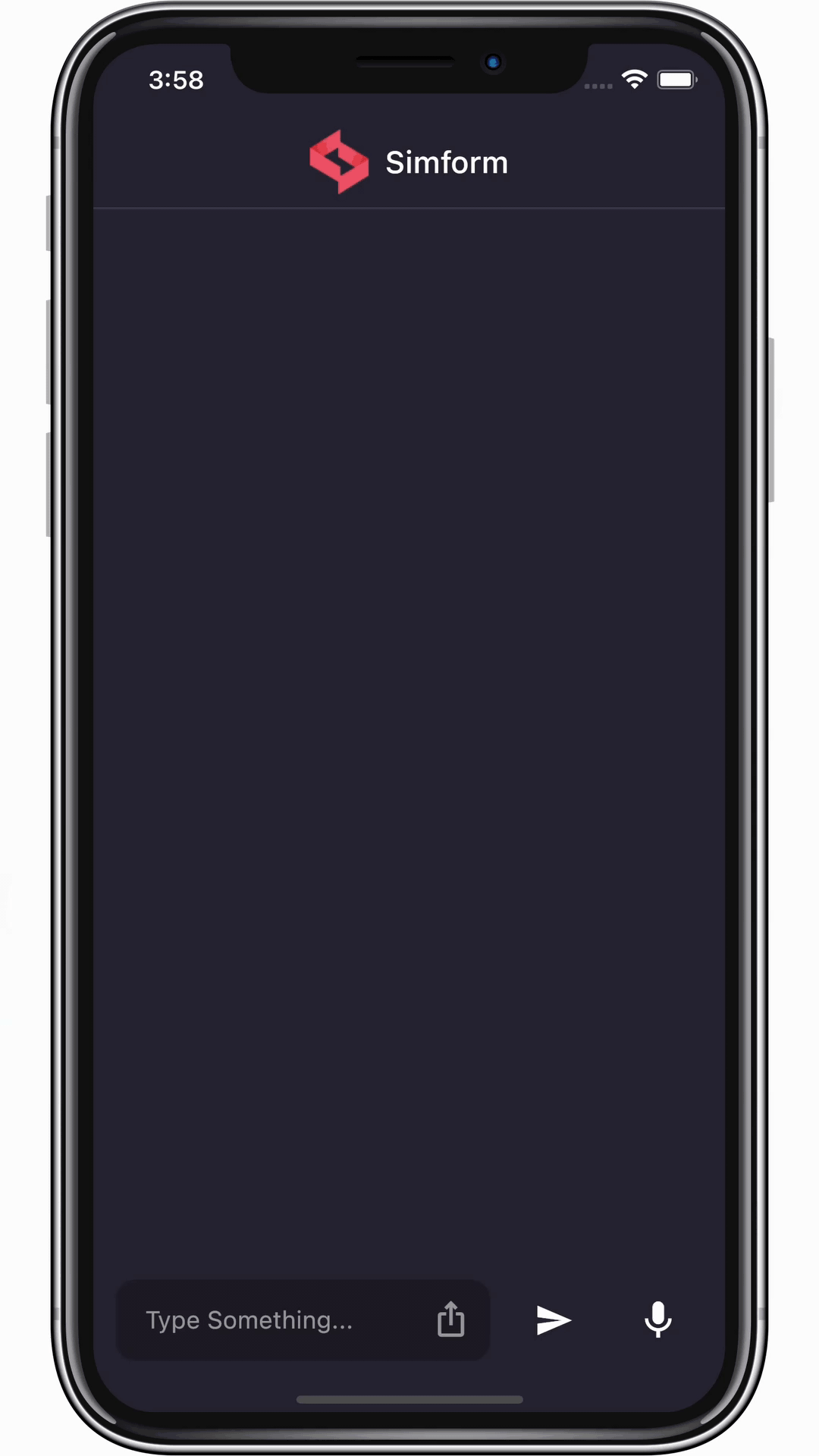
**Check [migration guide](https://github.com/SimformSolutionsPvtLtd/audio_waveforms/blob/main/migration_guide.md) to migrate from 0.1.5+1 to 1.0.0**
## Recorder
### Platform specific configuration
**Android**
Change the minimum Android sdk version to 21 (or higher) in your `android/app/build.gradle` file.
```
minSdkVersion 21
```
Add RECORD_AUDIO permission in `AndroidManifest.xml`
```
```
**IOS**
Add this two rows in `ios/Runner/Info.plist`
```
NSMicrophoneUsageDescription
This app requires Mic permission.
```
This plugin requires ios 10.0 or higher. So add this line in `Podfile`
```
platform :ios, '10.0'
```
**Installing**
1. Add dependency to `pubspec.yaml`
```dart
dependencies:
audio_waveforms:
```
*Get the latest version in the 'Installing' tab on [pub.dev](https://pub.dev/packages/audio_waveforms)*
2. Import the package.
```dart
import 'package:audio_waveforms/audio_waveforms.dart';
```
### Usage
1. Recording audio
```dart
RecorderController controller = RecorderController(); // Initialise
await controller.record(path: 'path'); // Record (path is optional)
final hasPermission = await controller.checkPermission(); // Check mic permission (also called during record)
await controller.pause(); // Pause recording
final path = await controller.stop(); // Stop recording and get the path
controller.refresh(); // Refresh waveform to original position
controller.dispose(); // Dispose controller
```
2. Use `AudioWaveforms` widget in widget tree
```dart
AudioWaveforms(
size: Size(MediaQuery.of(context).size.width, 200.0),
recorderController: controller,
enableGesture: true,
waveStyle: WaveStyle(
...
color: Colors.white,
showDurationLabel: true,
spacing: 8.0,
showBottom: false,
extendWaveform: true,
showMiddleLine: false,
gradient: ui.Gradient.linear(
const Offset(70, 50),
Offset(MediaQuery.of(context).size.width / 2, 0),
[Colors.red, Colors.green],
),
...
),
),
```
**Advance usage**
```dart
controller.updateFrequency = const Duration(milliseconds: 100); // Update speed of new wave
controller.androidEncoder = AndroidEncoder.aac; // Changing android encoder
controller.androidOutputFormat = AndroidOutputFormat.mpeg4; // Changing android output format
controller.iosEncoder = IosEncoder.kAudioFormatMPEG4AAC; // Changing ios encoder
controller.sampleRate = 44100; // Updating sample rate
controller.bitRate = 48000; // Updating bitrate
controller.onRecorderStateChanged.listen((state){}); // Listening to recorder state changes
controller.onCurrentDuration.listen((duration){}); // Listening to current duration updates
controller.onRecordingEnded.listen((duration)); // Listening to audio file duration
controller.recordedDuration; // Get recorded audio duration
controller.currentScrolledDuration; // Current duration position notifier
```
## Player
### Usage
```dart
PlayerController controller = PlayerController(); // Initialise
// Extract waveform data
final waveformData = await controller.extractWaveformData(
path: 'path',
noOfSamples: 100,
);
// Or directly extract from preparePlayer and initialise audio player
await controller.preparePlayer(
path: 'path',
shouldExtractWaveform: true,
noOfSamples: 100,
volume: 1.0,
);
await controller.startPlayer(finishMode: FinishMode.stop); // Start audio player
await controller.pausePlayer(); // Pause audio player
await controller.stopPlayer(); // Stop audio player
await controller.setVolume(1.0); // Set volume level
await controller.seekTo(5000); // Seek audio
final duration = await controller.getDuration(DurationType.max); // Get duration of audio player
controller.updateFrequency = UpdateFrequency.low; // Update reporting rate of current duration.
controller.onPlayerStateChanged.listen((state) {}); // Listening to player state changes
controller.onCurrentDurationChanged.listen((duration) {}); // Listening to current duration changes
controller.onCurrentExtractedWaveformData.listen((data) {}); // Listening to latest extraction data
controller.onExtractionProgress.listen((progress) {}); // Listening to extraction progress
controller.onCompletion.listen((_){}); // Listening to audio completion
controller.stopAllPlayer(); // Stop all registered audio players
controller.dispose(); // Dispose controller
```
**Use `AudioFileWaveforms` widget in widget tree**
```dart
AudioFileWaveforms(
size: Size(MediaQuery.of(context).size.width, 100.0),
playerController: controller,
enableSeekGesture: true,
waveformType: WaveformType.long,
waveformData: [],
playerWaveStyle: const PlayerWaveStyle(
fixedWaveColor: Colors.white54,
liveWaveColor: Colors.blueAccent,
spacing: 6,
...
),
...
);
```
## Main Contributors
近期下载者:
相关文件:
收藏者: