# news-app
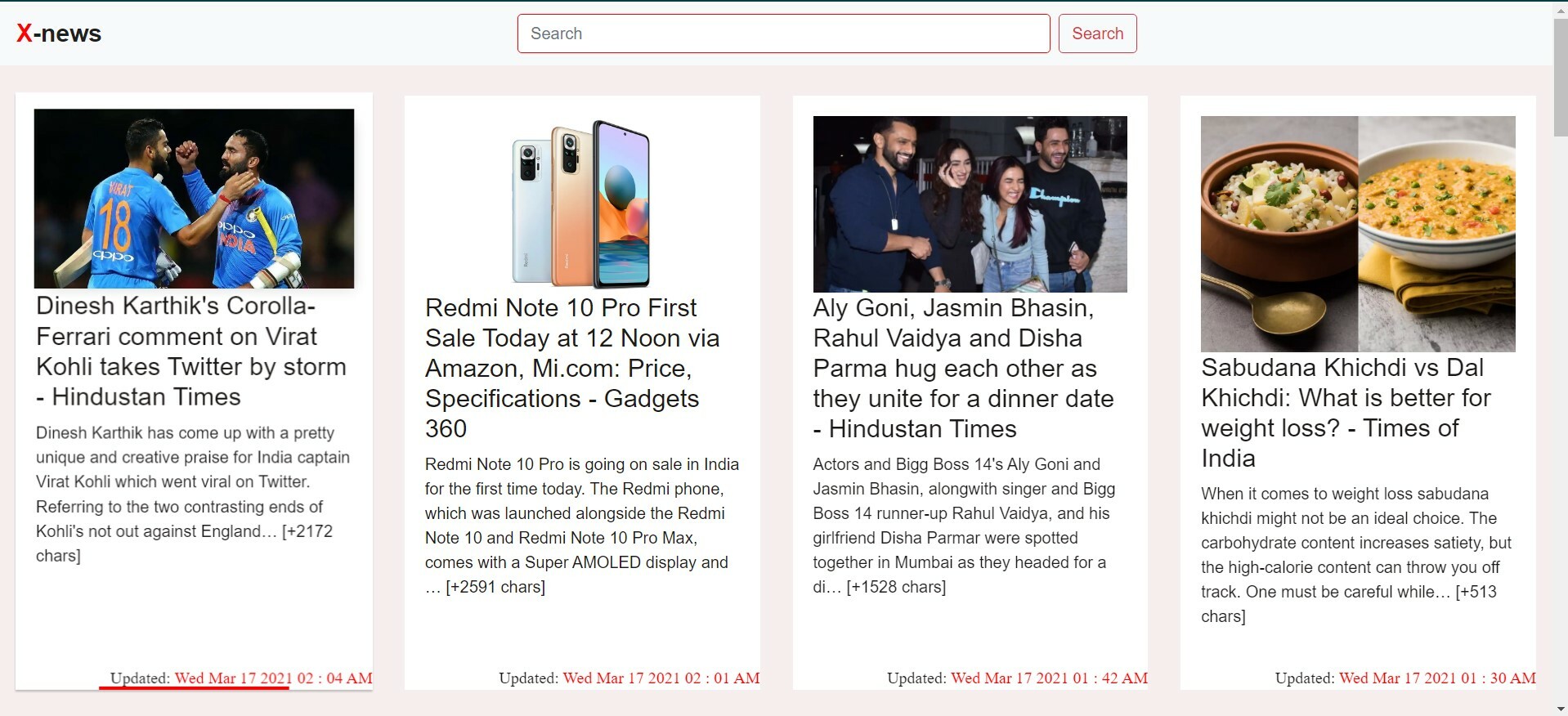
Today we are going to develop a simple News app with the help of NodeJS , Express , EJS and bootstrap.
There are going to be 2 main features in this website search and display news articles And we are going to use Newapi for getting news articles.
## Lets Start
### Initialize the New Project
To initialize the new project you just need to create a new folder _"News App"_ and open folder in visual studio code or any other IDE and run the below code in command line
```javascript
npm init
```
This takes only few seconds and also asks few question about your project like project name , description etc. after that initialization is over and a file name _"Package.json"_ is generated .
### Structure of project
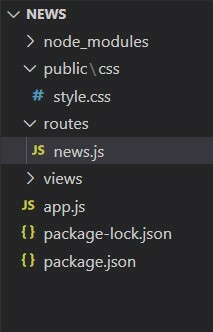
As with the reference of the above image create folders and files leave node_modules package-lock and package-json as they generate automatically .
### Install Dependencies
These are the Dependencies we need to install for our project.
```
express
ejs
body-parser
axios
math
moment
```
For installing these Dependencies you just need to write the below code in your terminal
```javascript
npm install express ejs body-parser axios math moment
```
### Setup App for run
To start the server automatically we just need to install Nodemon which restart server automatically when any change is detected
```javascript
npm install -D nodemon
```
Setup application for developer run and normal run. Just change the Script section with the below code in `package.json`.
```
"scripts": {
"start": "node app.js",
"dev": "nodemon app.js"
},
```
### Start developer local server
To start our app for testing/developer just simply type the following command in the command line:
```javascript
npm dev
```
### Application
Lets code our `app.js` file this is the main file and it will sit in the root of our website.
In this file we have to setup our server .
file:-> `app.js`
```javascript
const express = require('express')
const app=express()
const port = process.env.PORT||3000;
const bodyParser = require('body-parser');
const moment = require('moment')
app.locals.moment = moment;
// template engine
app.use(express.static('public'))
app.set('view engine','ejs')
app.use(bodyParser.urlencoded({ extended: true }));
app.use('/',require('./routes/news'))
app.set('views','./views')
app.listen(port,()=> console.log("started"))
```
### Routes
Lets build a extremal routes
External routing is a way of structuring your code so that it stays nice and organized by taking the route implementations outside of the main server file and moving them into a separate router file.
First you need your NewsApi API key for getting data , Go to [NewsApi](https://newsapi.org/) site and get your Api key then and simply replace `YOUR_API` in the url with your Api key in the below code
file:-> `routes/news.js`
```javascript
const express = require('express')
const axios = require('axios')
const newsr=express.Router()
const moment = require('moment')
const math = require('math')
newsr.get('/',async(req,res)=>{
try {
var url = 'http://newsapi.org/v2/top-headlines?' +
'country=in&' +
'apiKey=2c6bfa81c2e8403da6eff5d85b8d1432';
const news_get =await axios.get(url)
res.render('news',{articles:news_get.data.articles})
} catch (error) {
if(error.response){
console.log(error)
}
}
})
newsr.post('/search',async(req,res)=>{
const search=req.body.search
// console.log(req.body.search)
try {
var url = `http://newsapi.org/v2/everything?q=${search}&apiKey=2c6bfa81c2e8403da6eff5d85b8d1432`
const news_get =await axios.get(url)
res.render('news',{articles:news_get.data.articles})
} catch (error) {
if(error.response){
console.log(error)
}
}
})
module.exports=newsr
```
### Views
file:-> `views/news.ejs`
```javascript
News