tutorials
所属分类:文章/文档
开发工具:Shell
文件大小:0KB
下载次数:0
上传日期:2023-08-03 06:16:46
上 传 者:
sh-1993
说明: 图105:以编程方式访问上下文,
(FIWARE 105: Accessing Context Programmatically,)
文件列表:
.dockerignore (27, 2023-08-15)
.env (237, 2023-08-15)
FIWARE Accessing Context.postman_collection.json (32970, 2023-08-15)
LICENSE (1085, 2023-08-15)
docker-compose.yml (3636, 2023-08-15)
import-data (14669, 2023-08-15)
services (3662, 2023-08-15)
[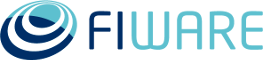](https://www.fiware.org/developers)
[](https://fiware-ges.github.io/orion/api/v2/stable/)
[](https://github.com/FIWARE/catalogue/blob/master/core/README.md)
[](https://opensource.org/licenses/MIT)
[](https://stackoverflow.com/questions/tagged/fiware)
[](https://fiware-tutorials.rtfd.io)
This tutorial teaches FIWARE users how to alter the context programmatically. The tutorial builds on the entities
created in the previous [stock management example](https://github.com/FIWARE/tutorials.Context-Providers/) and enables a
user understand how to write code in an [NGSI-v2](https://fiware.github.io/specifications/OpenAPI/ngsiv2) capable
[Node.js](https://nodejs.org/) [Express](https://expressjs.com/) application in order to retrieve and alter context
data. This removes the need to use the command-line to invoke cUrl commands.
The tutorial is mainly concerned with discussing code written in Node.js, however some of the results can be checked by
making [cUrl](https://ec.haxx.se/) commands.
[Postman documentation](https://fiware.github.io/tutorials.Accessing-Context/) for the same commands is also available.
[](https://app.getpostman.com/run-collection/fb5f564d9bc65fc3690e)
[](https://gitpod.io/#https://github.com/FIWARE/tutorials.Accessing-Context/tree/NGSI-v2)
- このチュートリアルは[日本語](README.ja.md)でもご覧いただけます。
## Contents
Details
- [Accessing the Context Data](#accessing-the-context-data)
- [Making HTTP requests in the language of your choice](#making-http-requests-in-the-language-of-your-choice)
- [Generating NGSI API Clients](#generating-ngsi-api-clients)
- [The teaching goal of this tutorial](#the-teaching-goal-of-this-tutorial)
- [Entities within a stock management system](#entities-within-a-stock-management-system)
- [Architecture](#architecture)
- [Prerequisites](#prerequisites)
- [Docker](#docker)
- [Cygwin](#cygwin)
- [Start Up](#start-up)
- [Stock Management Frontend](#stock-management-frontend)
- [NGSI v2 npm library](#ngsi-v2-npm-library)
- [Analyzing the Code](#analyzing-the-code)
- [Initializing the library](#initializing-the-library)
- [Reading Store Data](#reading-store-data)
- [Aggregating Products and Inventory Items](#aggregating-products-and-inventory-items)
- [Updating Context](#updating-context)
- [Next Steps](#next-steps)
# Accessing the Context Data
For a typical smart solution you will be retrieving context data from diverse sources (such as a CRM system, social
networks, mobile apps or IoT sensors for example) and then analyzing the context programmatically to make appropriate
business logic decisions. For example in the stock management demo, the application will need to ensure that the prices
paid for each item always reflect the current price held within the **Product** entity. For a dynamic system, the
application will also need to be able to amend the current context. (e.g. creating or updating data or actuating a
sensor for example)
In general terms, three basic scenarios are defined below:
- Reading Data - e.g. Give me all the data for the **Store** entity `urn:ngsi-ld:Store:001`
- Aggregation - e.g. Combine the **InventoryItems** entities for Store `urn:ngsi-ld:Store:001` with the names and
prices of the **Product** entities for sale
- Altering the Context - e.g. Make a sale of a product:
- Update the daily sales records by the price of the **Product**
- decrement the `shelfCount` of the **InventoryItem** entity
- Create a new Transaction Log record showing the sale has occurred
- Raise an alert in the warehouse if less than 10 objects remain on sale
- etc.
As you can see the business logic behind each request to access/amend context can range from the simple to complex
depending upon business needs.
## Making HTTP Requests in the language of your choice
The [NGSI-v2](https://fiware.github.io/specifications/OpenAPI/ngsiv2) specification defines a language agnostic REST API
based on the standard usage of HTTP verbs. Therefore context data can be accessed by any programming language, simply
through making HTTP requests.
Here for example is the same HTTP request written in [PHP](https://secure.php.net/), [Node.js](https://nodejs.org/) and
[Java](https://www.oracle.com/java/)
#### PHP (with `HTTPRequest`)
```php
setUrl('http://localhost:1026/v2/entities/urn:ngsi-ld:Store:001');
$request->setMethod(HTTP_METH_GET);
$request->setQueryData(array(
'options' => 'keyValues'
));
try {
$response = $request->send();
echo $response->getBody();
} catch (HttpException $ex) {
echo $ex;
}
```
#### Node.js (with `request` library)
```javascript
const request = require('request');
const options = {
method: 'GET',
url: 'http://localhost:1026/v2/entities/urn:ngsi-ld:Store:001',
qs: { options: 'keyValues' }
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
```
#### Java (with `CloseableHttpClient` library)
```java
CloseableHttpClient httpclient = HttpClients.createDefault();
try {
HttpGet httpget = new HttpGet("http://localhost:1026/v2/entities/urn:ngsi-ld:Store:001?options=keyValues");
ResponseHandler
responseHandler = new ResponseHandler() {
@Override
public String handleResponse(
final HttpResponse response) throws ClientProtocolException, IOException {
int status = response.getStatusLine().getStatusCode();
if (status >= 200 && status < 300) {
HttpEntity entity = response.getEntity();
return entity != null ? EntityUtils.toString(entity) : null;
} else {
throw new ClientProtocolException("Unexpected response status: " + status);
}
}
};
String body = httpclient.execute(httpget, responseHandler);
System.out.println(body);
} finally {
httpclient.close();
}
```
## Generating NGSI API Clients
As you can see from the examples above, each one uses their own programming paradigm to do the following:
- Create a well formed URL.
- Make an HTTP GET request.
- Retrieve the response.
- Check for an error status and throw an exception if necessary.
- Return the body of the request for further processing.
Since such boilerplate code is frequently re-used it is usually hidden within a library.
The [`swagger-codegen`](https://github.com/swagger-api/swagger-codegen) tool is able to generate boilerplate API client
libraries in a wide variety of programming languages directly from the
[NGSI v2 Swagger Specification](https://fiware.github.io/specifications/OpenAPI/ngsiv2). Currently `swagger-codegen`
will generate code for the following languages:
- ActionScript, Ada, Apex, Bash, C#, C++, Clojure, Dart, Elixir, Elm, Eiffel, Erlang, Go, Groovy, Haskell, Java,
Kotlin, Lua, Node.js, Objective-C, Perl, PHP, PowerShell, Python, R, Ruby, Rust, Scala, Swift, TypeScript
For example the command:
```console
swagger-codegen generate \
-l javascript \
-i https://fiware.github.io/specifications/OpenAPI/ngsiv2/ngsiv2-openapi.json
```
Will generate a default ES5 npm package for NGSI v2 directly from the current specification.
Additional information can be found by running
```console
swagger-codegen help generate
```
With information about the customization switches available for a specific language found by running
```console
swagger-codegen config-help -l
```
## The teaching goal of this tutorial
The aim of this tutorial is to improve developer understanding of programmatic access of context data through defining
and discussing a series of generic code examples covering common data access scenarios. For this purpose a simple
Node.js Express application will be created.
The intention here is not to teach users how to write an application in Express - indeed any language could have been
chosen. It is merely to show how **any** sample programming language could be used alter the context to achieve the
business logic goals.
Obviously, your choice of programming language will depend upon your own business needs - when reading the code below
please keep this in mind and substitute Node.js with your own programming language as appropriate.
## Entities within a stock management system
The relationship between our entities is defined as shown:
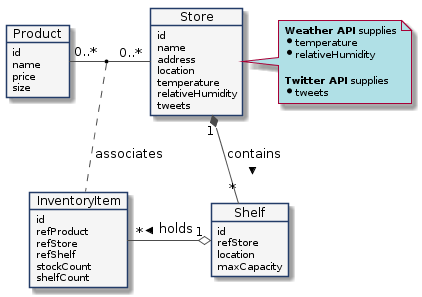
The **Store**, **Product** and **InventoryItem** entities will be used to display data on the frontend of our demo
application.
# Architecture
This application will make use of only one FIWARE component - the
[Orion Context Broker](https://fiware-orion.readthedocs.io/en/latest/). Usage of the Orion Context Broker is sufficient
for an application to qualify as _“Powered by FIWARE”_.
Currently, the Orion Context Broker relies on open source [MongoDB](https://www.mongodb.com/) technology to keep
persistence of the context data it holds. To request context data from external sources, a simple Context Provider NGSI
proxy has also been added. To visualize and interact with the Context we will add a simple Express application
Therefore, the architecture will consist of four elements:
- The [Orion Context Broker](https://fiware-orion.readthedocs.io/en/latest/) which will receive requests using
[NGSI-v2](https://fiware.github.io/specifications/OpenAPI/ngsiv2)
- The underlying [MongoDB](https://www.mongodb.com/) database :
- Used by the Orion Context Broker to hold context data information such as data entities, subscriptions and
registrations
- The **Context Provider NGSI** proxy which will:
- receive requests using [NGSI-v2](https://fiware.github.io/specifications/OpenAPI/ngsiv2)
- makes requests to publicly available data sources using their own APIs in a proprietary format
- returns context data back to the Orion Context Broker in
[NGSI-v2](https://fiware.github.io/specifications/OpenAPI/ngsiv2) format.
- The **Stock Management Frontend** which will:
- Display store information
- Show which products can be bought at each store
- Allow users to "buy" products and reduce the stock count.
Since all interactions between the elements are initiated by HTTP requests, the entities can be containerized and run
from exposed ports.
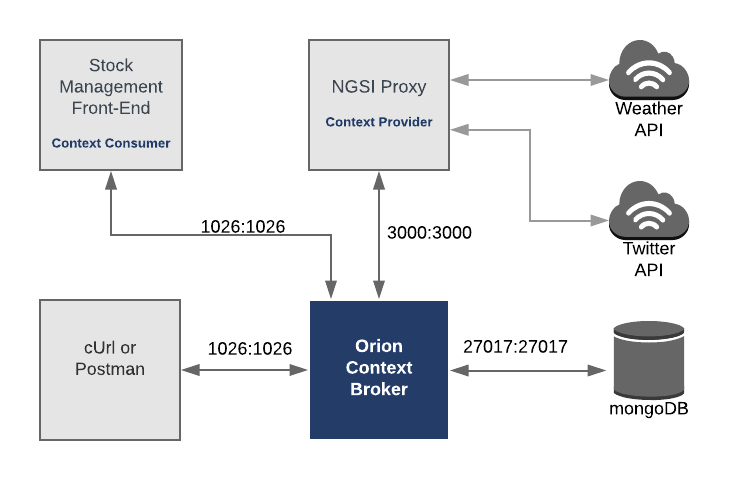
The necessary configuration information can be seen in the services section of the associated `docker-compose.yml` file.
It has been described in a [previous tutorial](https://github.com/FIWARE/tutorials.Context-Providers/)
# Prerequisites
## Docker
To keep things simple both components will be run using [Docker](https://www.docker.com). **Docker** is a container
technology which allows to different components isolated into their respective environments.
- To install Docker on Windows follow the instructions [here](https://docs.docker.com/docker-for-windows/)
- To install Docker on Mac follow the instructions [here](https://docs.docker.com/docker-for-mac/)
- To install Docker on Linux follow the instructions [here](https://docs.docker.com/install/)
**Docker Compose** is a tool for defining and running multi-container Docker applications. A
[YAML file](https://raw.githubusercontent.com/Fiware/tutorials.Entity-Relationships/master/docker-compose.yml) is used
configure the required services for the application. This means all container services can be brought up in a single
command. Docker Compose is installed by default as part of Docker for Windows and Docker for Mac, however Linux users
will need to follow the instructions found [here](https://docs.docker.com/compose/install/)
You can check your current **Docker** and **Docker Compose** versions using the following commands:
```console
docker-compose -v
docker version
```
Please ensure that you are using Docker version 20.10 or higher and Docker Compose 1.29 or higher and upgrade if
necessary.
## Cygwin
We will start up our services using a simple bash script. Windows users should download [cygwin](http://www.cygwin.com/)
to provide a command-line functionality similar to a Linux distribution on Windows.
# Start Up
All services can be initialized from the command-line by running the bash script provided within the repository. Please
clone the repository and create the necessary images by running the commands as shown:
```console
git clone https://github.com/FIWARE/tutorials.Accessing-Context.git
cd tutorials.Accessing-Context
git checkout NGSI-v2
./services create; ./services start;
```
This command will also import seed data from the previous
[Stock Management example](https://github.com/FIWARE/tutorials.Context-Providers) on startup.
> :information_source: **Note:** If you want to clean up and start over again you can do so with the following command:
>
> ```console
> ./services stop
> ```
# Stock Management Frontend
All the code Node.js Express for the demo can be found within the `proxy` folder within the GitHub
repository.[Stock Management example](https://github.com/FIWARE/tutorials.NGSI-v2/tree/master/context-provider). The
application runs on the following URLs:
- `http://localhost:3000/app/store/urn:ngsi-ld:Store:001`
- `http://localhost:3000/app/store/urn:ngsi-ld:Store:002`
- `http://localhost:3000/app/store/urn:ngsi-ld:Store:003`
- `http://localhost:3000/app/store/urn:ngsi-ld:Store:004`
> :information_source: **Tip** Additionally, you can also watch the status of recent requests yourself by following the
> container logs or viewing information on `localhost:3000/app/monitor` on a web browser.
>
> 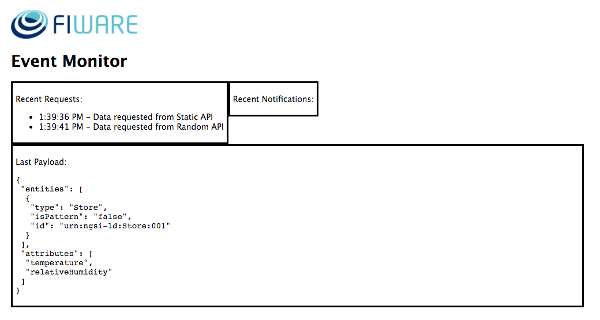
## NGSI v2 npm library
A Swagger Generated NGSI v2 client [npm library](https://github.com/smartsdk/ngsi-sdk-javascript) has been developed by
the [SmartSDK](https://www.smartsdk.eu/) team. This is a callback-based library which will be used to take care of our
low level HTTP requests and will simplify the code to be written. The methods exposed in the library map directly onto
the NGSI v2 [CRUD operations](https://github.com/FIWARE/tutorials.CRUD-Operations#what-is-crud) with the following
names:
| HTTP Verb | `/v2/entities` | `/v2/entities/` |
| ---------- | :--------------------------------------------------------------------------------------------------------------: | :------------------------------------------------------------------------------------------------------------------: |
| **POST** | [`createEntity()`](https://github.com/smartsdk/ngsi-sdk-javascript/blob/master/docs/EntitiesApi.md#createEntity) | :x: |
| **GET** | [`listEntities()`](https://github.com/smartsdk/ngsi-sdk-javascript/blob/master/docs/EntitiesApi.md#listEntities) | [`retrieveEntity()`](https://github.com/smartsdk/ngsi-sdk-javascript/blob/master/docs/EntitiesApi.md#retrieveEntity) |
| **PUT** | :x: | :x: |
| **PATCH** | :x: | :x: |
| **DELETE** | :x: | [`removeEntity()`](https://github.com/smartsdk/ngsi-sdk-javascript/blob/master/docs/EntitiesApi.md#removeEntity) |
| HTTP Verb | `.../attrs` | `.../attrs/` | `.../attrs//value` |
| ----------- | :--------------------------------------------------------------------------------------------------------------------------------------------------: | :------------------------: | :------------------------------------------------------------------------------------------------------------------------------------: |
| **POST** | [`updateOrAppendEntityAttributes()`](https://github.com/smartsdk/ngsi-sdk-javascript/blob/master/docs/EntitiesApi.md#updateOrAppendEntityAttributes) | :x: | :x: |
| **GET** | [`retrieveEntityAttributes()`](https://github.com/smartsdk/ngsi-sdk-javascript/blob/master/docs/EntitiesApi.md#retrieveEntityAttributes) | :x: | [`getAttributeValue()`](https://github.com/smartsdk/ngsi-sdk-javascript/blob/master/docs/AttributeValueApi.md#getAttributeValue) |
| **PUT** | :x: | :x: | [`updateAttributeValue()`](https://github.com/smartsdk/ngsi-sdk-javascript/blob/master/docs/AttributeValueApi.md#updateAttributeValue) |
| **PATCH** | [`updateExistingEntityAttributes()`](https://github.com/smartsdk/ngsi-sdk-javascript/blob/master/docs/EntitiesApi.md#updateExistingEntityAttributes) | :x: | :x: |
| **DELETE**. | :x: | `removeASingleAttribute()` | :x: |
## Analyzing the Code
The code under discussion can be found within the `store` controller in the
[Git Repository](https://github.com/FIWARE/tutorials.NGSI-v2/blob/master/context-provider/controllers/ngsi-v2/store.js)
### Initializing the library
We don't want to reinvent the wheel and spend time writing a unnecessary boilerplate code for HTTP access. Therefore we
will use the existing `ngsi_v2` npm librar ... ...
近期下载者:
相关文件:
收藏者: